IR-remote I build from scratch back in 9th grade for trolling teachers :)
The “remoteconTROLL” is based around an Arduino nano wired to a few buttons and an infrared LED. the individual buttons are mapped to different hexadecimal codes which are then send via the LED. For getting hold of the codes i also build a simple IR-code-reader.
I build this simple project in 9th grade for trolling my teachers by messing with the settings etc. of the beamers during a lesson. Regarding this I can tell that no teacher even had the slightest idea that someone was capable of cloning the remotecontrols…
In the end it got a little boring anyway after a few times using it…
Details
-
Power supply: 9V Battery
-
Range: ~ 5-10m
-
Features:
- 11 individual buttons
- 2 settings for 2 different types of beamers
- extension cable for the infrared LED
(for the remote not getting spottet so easily) - 2 IR-LEDs in parallel for a wider angle of range
- spam-mode sending random 1s & 0s
Finished remotecontrol
Components
amount | part |
---|---|
1x | perfboard |
1x | Arduino nano |
2x | IR-LED |
11x | tactile switches |
13x | 1.5k resistors |
2x | slide switch |
1x | 9V-batteryclip |
Course of action
Wiring
The wiring is quite simple. Each button is connected to a pin of the arduino which is pulled LOW (to GND) via a 1,5k pulldown-resistor. When pressing the button the pin is pulled HIGH (to 5V) which then is recognized by the arduino. Schematic. The circuit is then simply repeated for each button and soldered onto a perfboard.
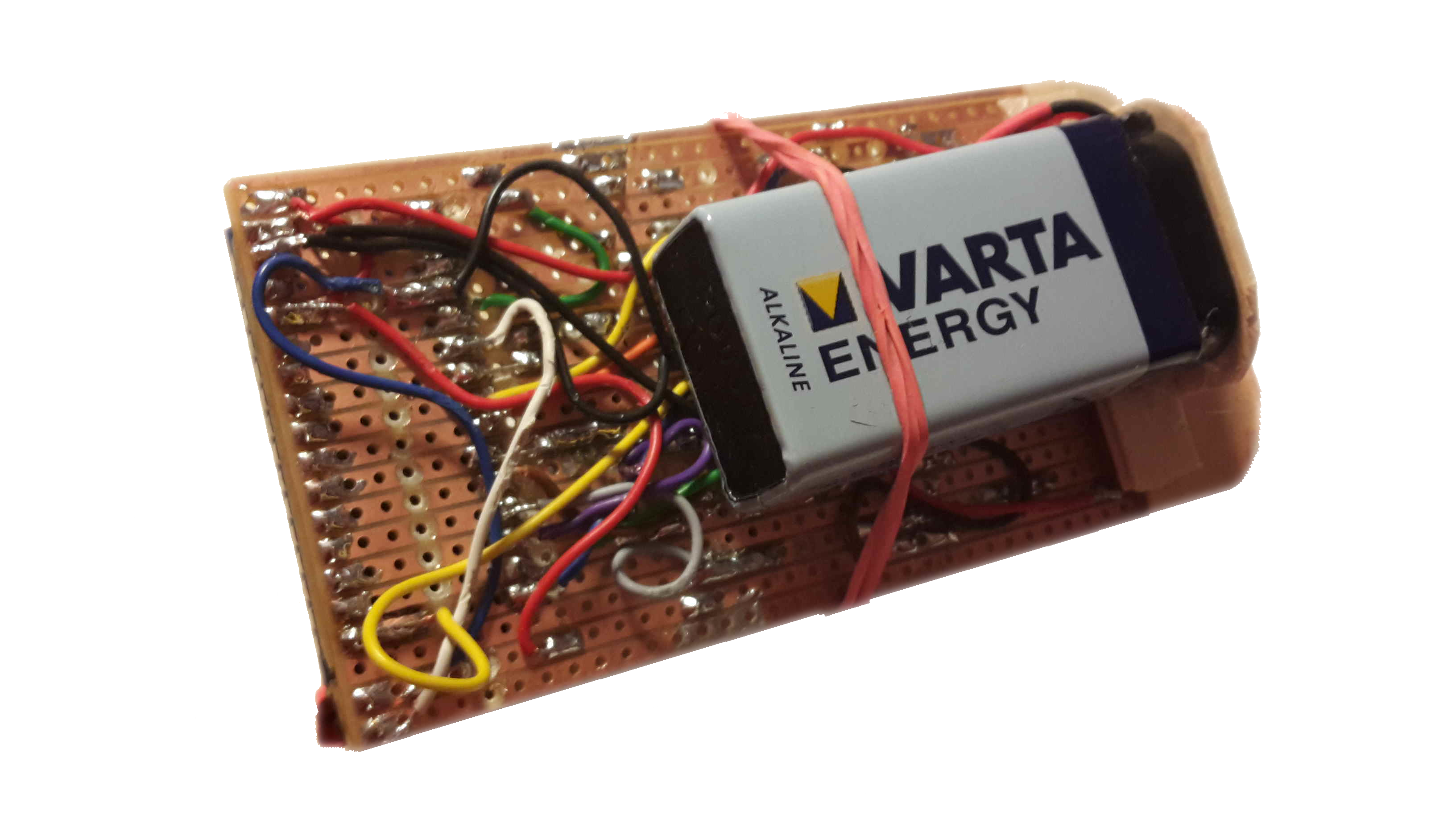
Programming
The Source-Code is quite simple. It utilizes the “IRremote” library and maps the individual buttons to the corresponding hexadecimal codes being send by the LED. Details for customizing the remotecontrol are explained within the sourcecode:
//********************************
//BY MORITZ RAMBOLD 2018
//********************************
//
//for different devices change the hexadecimal codes for each button to the ones of your device
#include <IRremote.h>
#include <OneButton.h>
#include <Bounce2.h>
IRsend irsend;
const int KeystoneUp = 2;
const int KeystoneDown = 12;
const int SVIDEO = 4;
const int VGA = 5;
const int ONOFF = 10;
const int AVSTUMM = 7;
const int ZOOM = 8;
const int BRIGHTNESS = 9;
const int FREEZE = 6;
const int SPAMBUTTON = 11; //outputs a series of 1s and 0s blocking use of other remotecontrols of the device
const int EpsonSwitch = 13; //selects between 2 different beamer models/devices
void setup() {
pinMode(KeystoneUp, INPUT);
pinMode(KeystoneDown, INPUT);
pinMode(SVIDEO, INPUT);
pinMode(VGA, INPUT);
pinMode(ONOFF, INPUT);
pinMode(AVSTUMM, INPUT);
pinMode(ZOOM, INPUT);
pinMode(BRIGHTNESS, INPUT);
pinMode(FREEZE, INPUT);
pinMode(SPAMBUTTON, INPUT);
pinMode(EpsonSwitch, INPUT);
}
void loop() {
if (digitalRead(KeystoneUp) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB3A15E, 32);
}
else if (digitalRead(KeystoneUp) == HIGH && digitalRead(EpsonSwitch) == LOW)
{}
if (digitalRead(KeystoneDown) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB321DE, 32);
}
else if (digitalRead(KeystoneDown) == HIGH && digitalRead(EpsonSwitch) == LOW)
{}
if (digitalRead(SVIDEO) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB3C13E, 32);
}
else if (digitalRead(SVIDEO) == HIGH && digitalRead(EpsonSwitch) == LOW) {
irsend.sendNEC(0xC1AA39C6, 32);
}
if (digitalRead(VGA) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB3718E, 32);
}
else if (digitalRead(VGA) == HIGH && digitalRead(EpsonSwitch) == LOW)
{}
if (digitalRead(ONOFF) == HIGH && digitalRead(EpsonSwitch) == HIGH) { //for Optoma
irsend.sendNEC(0x4CB3817E, 32);
}
else if (digitalRead(ONOFF) == HIGH && digitalRead(EpsonSwitch) == LOW) { //for Epson
irsend.sendNEC(0xC1AA09F6, 32);
}
if (digitalRead(AVSTUMM) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB351AE, 32);
}
else if (digitalRead(AVSTUMM) == HIGH && digitalRead(EpsonSwitch) == LOW) {
irsend.sendNEC(0xC1AAC936, 32);
}
if (digitalRead(ZOOM) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB3916E, 32);
}
else if (digitalRead(ZOOM) == HIGH && digitalRead(EpsonSwitch) == LOW)
{}
if (digitalRead(BRIGHTNESS) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB3E11E, 32);
}
else if (digitalRead(BRIGHTNESS) == HIGH && digitalRead(EpsonSwitch) == LOW)
{}
if (digitalRead(FREEZE) == HIGH && digitalRead(EpsonSwitch) == HIGH) {
irsend.sendNEC(0x4CB3D12E, 32);
}
else if (digitalRead(FREEZE) == HIGH && digitalRead(EpsonSwitch) == LOW) {
irsend.sendNEC(0xC1AA4906, 32);
}
if (digitalRead(SPAMBUTTON) == HIGH && digitalRead(EpsonSwitch) == HIGH) { // Universal spamsignal
irsend.sendNEC(0x01010101, 32);
}
else if (digitalRead(SPAMBUTTON) == HIGH && digitalRead(EpsonSwitch) == LOW) {
irsend.sendNEC(0x01010101, 32);
}
}